- Introduction
- Stage management and errors
- Trading and token evaluation
- Statistics
- Dockerizing
- Percentage strategy
- Winning pattern
- Solana congestion
- Telegram Bot
- Making money
- Discord bot
- Ending
- Conclusion
1. Introduction
If you’re reading this, you’re like us—already invested in cryptocurrencies and eyeing the next big thing in meme coins. This blog post is for those planning to start their own trading bot and dream of becoming a millionaire really fast! 😀 We won’t dive too deep into technical details, but we’ll share our journey and the lessons we learned. Plus, the really basic base script of our first stage is available on GitHub.
So, how did it all start?
With crypto prices soaring and meme coins making waves, I thought, “Why not build a trading bot?” I pitched the idea to my close friend Filip Djeze Bartus, and just like that, our adventure began.
Over the next six months, we poured hundreds and hundreds of hours into building this bot. We faced countless challenges and learned a ton along the way.
We hope our story provides valuable insights and helps you navigate the wild world of cryptocurrency trading. Happy trading, and may your bots be ever profitable! 🚀
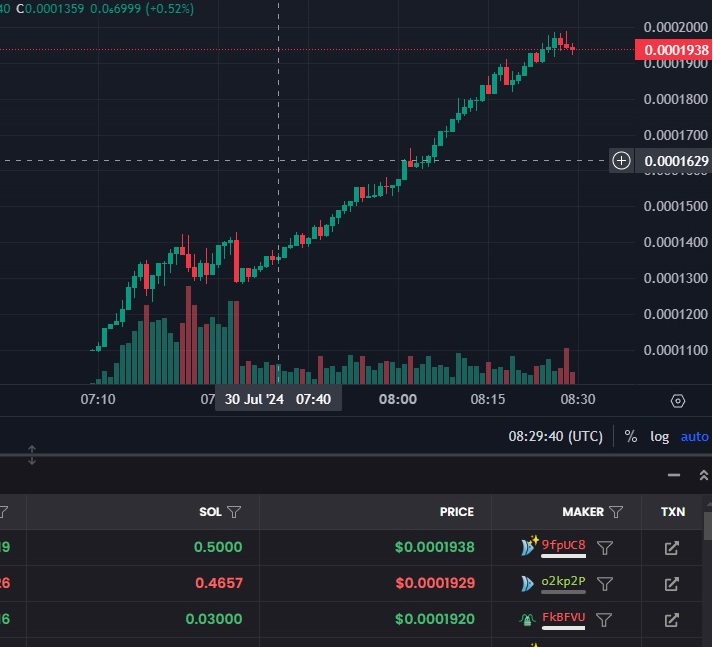
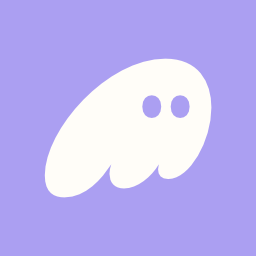
Since we had our sights set on memecoins and Solana having the smallest transaction fees, it was a no-brainer. We skipped the deep dive into research because we were already pretty familiar with Raydium, since we both tried day trading with bots on Raydium before. A quick peek at Raydium documentation and Solana’s, and we were off to the races. But first, we set up our Phantom wallet with multiple accounts. We opted for TypeScript in a Node.js environment because it’s what we knew best and, let’s be honest, npm has everything. With our setup in place, we were itching to start building.
With the project set up, it was time to get our hands dirty with some coding. The big question was, “Dude, should we start on a test network?” Naaah, let’s go straight to the mainnet—no time! 😄 So, mainnet it was.
Our first mission was connecting the wallet and validating our WSOL (Wrapped SOL) and SOL accounts. To keep things secure, we stored our private keys in a .env file and wrote a simple environment loader to pull them into our bot securely—no hardcoding or accidental GitHub leaks for us! With the keys loaded and some default constants like the Solana connection set up, we connected our wallet to the bot, making it our main entry point to the Solana blockchain. Before diving into trading, we made sure our wallet had enough funds by checking our SOL balance for transaction fees and validating our WSOL balance for trading. With those balances confirmed, we were ready to roll!
So how to discover new pools on Raydium DEX? We knew this was crucial for getting in early on potentially lucrative trades. We started by trying two different methods: subscribing to logs and monitoring account changes on an open Solana connection. This allowed us to keep an eye on the latest activities and spot new opportunities as they emerged. To enhance our discovery capabilities, we later added a Telegram bot scanner to our toolkit. This scanner monitored various crypto channels and alerted us to any promising new memecoins. But more on that exciting saga later!
Once we discovered a new pool, the next step was to create the necessary pool keys. There are various ways to achieve this, but we settled on fetching the pool account data and market account data to prepare the required pool keys. With the keys ready, we prepared a buy transaction message, sent it, and waited for confirmation.
For the selling process, our first step was to fetch the token balance from our wallet for the newly acquired token, ensuring we had the exact amount ready for sale. After confirming the balance, we retrieved the waiting time from our .env file, like we did for the buy amount, which dictated when the token should be sold. With this information in hand, we crafted the sell transaction message, mirroring the method used during the buy process. Once the transaction was prepared, we executed it and patiently awaited confirmation. This marked the completion of our first stage in the trading bot journey.
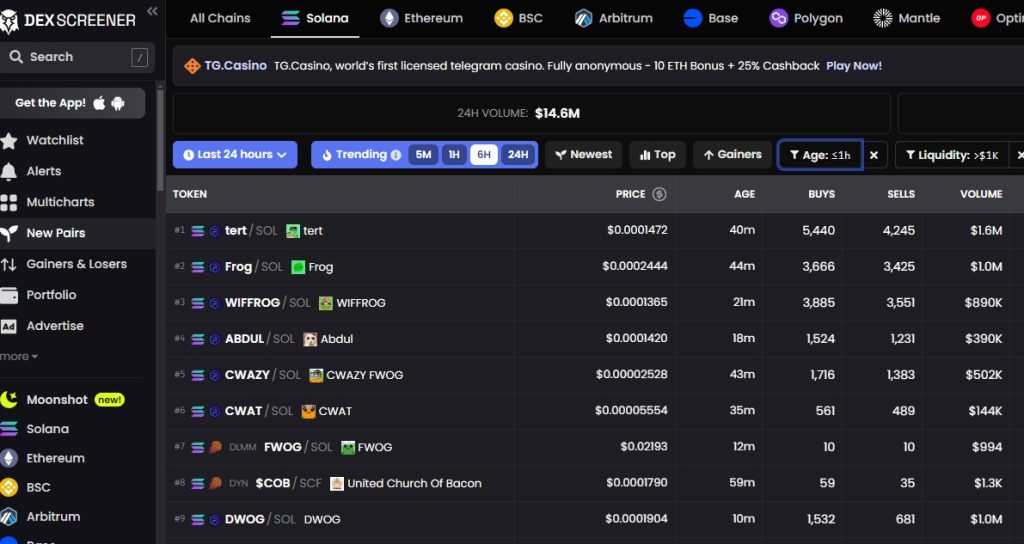
2. State management and errors
The next stage began with a lot of questions and bugs, revealing the inefficiencies in our initial code. Up until this point, everything had been developed on the fly. If we aimed to scale our operation, a more systematic approach was necessary. We implemented state management and introduced shared global constants, variables, and classes.
We encountered numerous errors during our fetch operations, such as failures in retrieving block hashes, confirmation issues, and market information failures. To address these challenges, we implemented robust error handling strategies across our codebase. Every fetch method was wrapped in a try-catch statement with retry mechanisms to handle transient failures gracefully.
In our critical buy and sell transactions, we implemented special stage error handling to swiftly detect and address errors without delays. This was particularly valuable during selling transactions, as we were determined not to hold onto unwanted tokens in our wallets! 😀
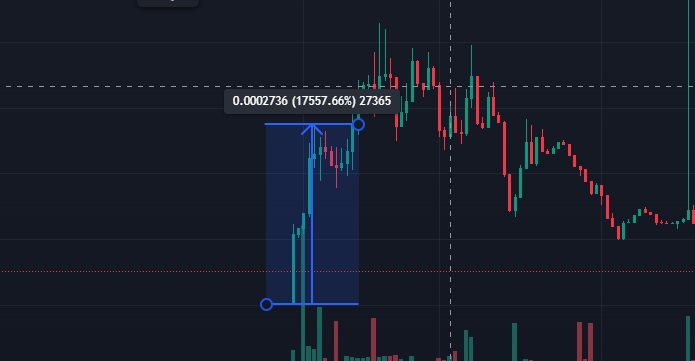
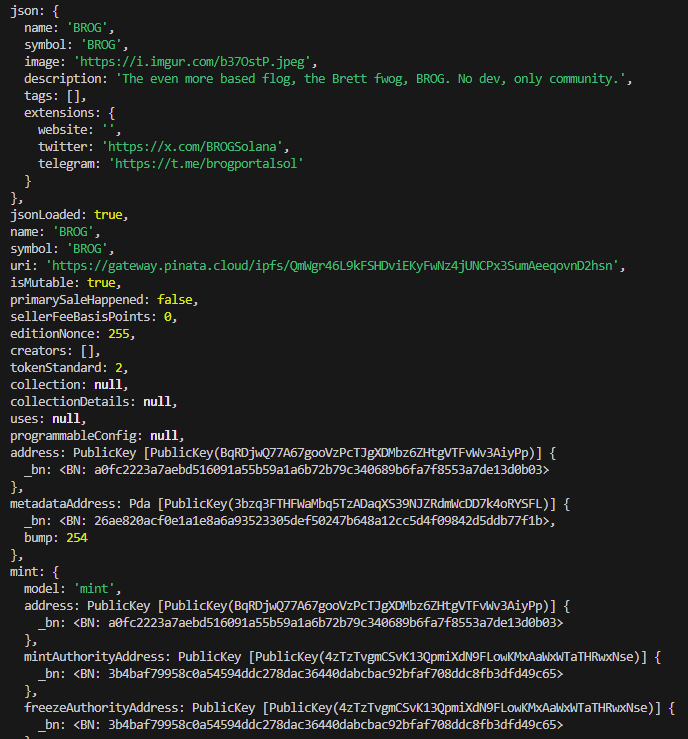
3. Trading and token evaluation
Since we started as a true sniper bot, we grabbed every token we stumbled upon—yep, bought ’em all – discovered them, and bought 😄. Our original plan was to sell them after a certain time, like within a minute of buying, because all tokens pump like crazy at the beginning, right? How did that turn out? Well, turns out, it’s not that simple. Finding a new pool takes time, and getting our transactions on the blockchain added even more delay. Our quickest was 33 seconds, but by the time we tried to sell it, it could easily hit the 2-minute mark .And in that timeframe, scammers could’ve already drained the pool dry, leaving us in the red. So what did we do? First, we considered rewriting everything in Rust to optimize and gain speed. But instead, we decided to first start with token evaluation.
The easiest way for scammers to mess with your bot is by having the freeze authority and mint authority enabled on their tokens. In the first case, the bot buys the token, but only a specific wallet address (provided by the token owner) can sell it, leaving you stuck. Can’t believe such tokens make it to the market? Trust us, they do. In the second case, if the mint authority is enabled, the token owner can mint new tokens at will, easily sell them, and drain the pool’s liquidity.
So, we started fetching token information. We didn’t stop at these two simple checks. We built an entire class for a comprehensive token evaluation process. Nothing was hardcoded; we could select what to check for in a token via .env file settings. There are two types of evaluations: strict and score. The strict setting goes through each required token attribute to see if it’s true or false. The score setting sums points for token attributes, and if the score exceeds the threshold set by the user, it buys the token. We could set points for each attribute based on its importance. Some settings we could configure include checking if the token has: mint authority, freeze authority, a description, an image, a website, a Telegram, a X(Twitter)…
4. Statistics
“Dude, how are we going to handle all the statistics? We can’t just keep looking at the wallet anymore—there are too many transactions and token evaluations. And we hate Excel. So, what did we do? We added a PostgreSQL database and linked it to Directus, a simple yet powerful headless CMS.
This added a whole new level of complexity to our project but made our lives so much easier for handling statistics. We connected all our current logged data to the database and added numerous new variables for tracking every statistic that came to mind. One of the most important features, besides the statistics, was saving discovered pools to a shared database. This meant that if my bot found a pool before Filip’s bot, it would already be in the database and traded only once.
After setting up Directus with a graphical interface showing all the data, we were ready for massive testing… or were we?”
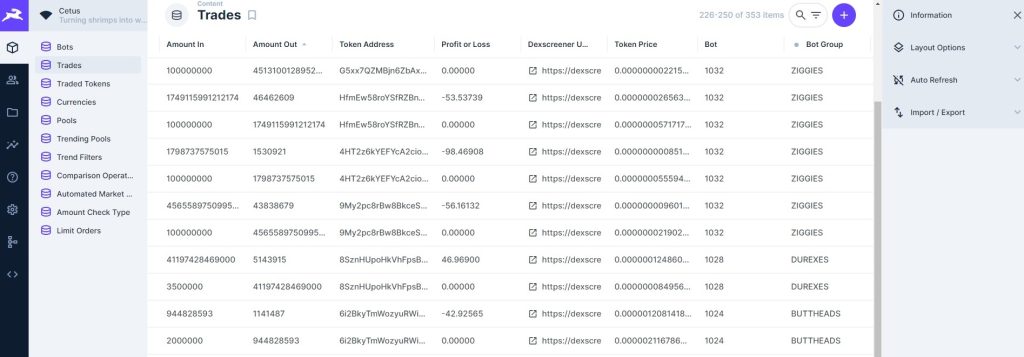
5. Dockerizing
Since we were running our bot locally and constantly developing new features, we hit a new snag: Solana connection timeouts due to too many requests. We didn’t want to rely on external providers like QuickNode, so we set up a Docker container to have the bot connect over a VPN. This allowed us to run the bot and develop new features simultaneously without the same IP causing conflicts.
Using the VPN, we connected from locations all over the world. One big advantage was getting closer to the parent nodes of the Solana blockchain. For instance, if we ran the bot in North America and the parent node was also in North America, our bot would recognize new pools faster than our bot in Europe. We were basically running a mini botnet, optimizing our chances. Now, all that’s left is to make some money! 😄
6. Percentage strategy
We were running our whole botnet 24/7 with different token evaluations and… nothing. We simply weren’t turning a profit. Selling after a set time was a total failure. Sure, you might make 500% on one token, but then the next 9 tokens would give you a 100% loss each. So, we added a Decide Action State class to level up our strategy.
This class was designed to calculate the token price at the time of purchase and then read user settings to decide whether to sell at a certain profit percentage or a certain loss percentage.The Decide Action State class would continuously monitor the token’s price after purchase, comparing it against the user-defined thresholds for profit and loss. If the price hit the desired profit level, the bot would execute a sell order. Conversely, if the price dropped to the specified loss level, the bot would cut its losses and sell to avoid further damage.
We didn’t use any external API for token prices. Instead, we calculated the token price at the time of purchase based on our wallet’s token balance and the amount of WSOL spent. For the current token price, we fetched pool data and calculated the price based on the provided pool liquidity. Our calculations were based on the Constant Product Curve and Offset Curve Concept.
Figuring all this out took some time, especially since JavaScript’s 64-bit integers weren’t sufficient for our needs. We had to bring in the BN (BigNumber) library to handle the large integer calculations accurately. This library allowed us to work with arbitrarily large numbers and perform precise arithmetic operations necessary for our bot’s calculations. Soooooo many numbers
7. Winning pattern
So now we’re running the whole botnet with a percentage strategy, and interestingly, some of the bots showed promise. But sadly, the same story as before: even if they were promising they didn’t turn a profit.. Despite testing different strategies and tweaking our token evaluation criteria, the bot was still in the red.
How did we deal with these scammers that kept making us lose money? We went nuts with token metadata evaluation. We added tons more details: description length, where the images were hosted, whether the token had a creator attribute, if the token was created on a specific website…
Then we finally found a pattern. If the token JSON and token image were uploaded to https://ipfs.io/ipfs, our stats showed that we were making money. Finally, hard work paying off, or is it?
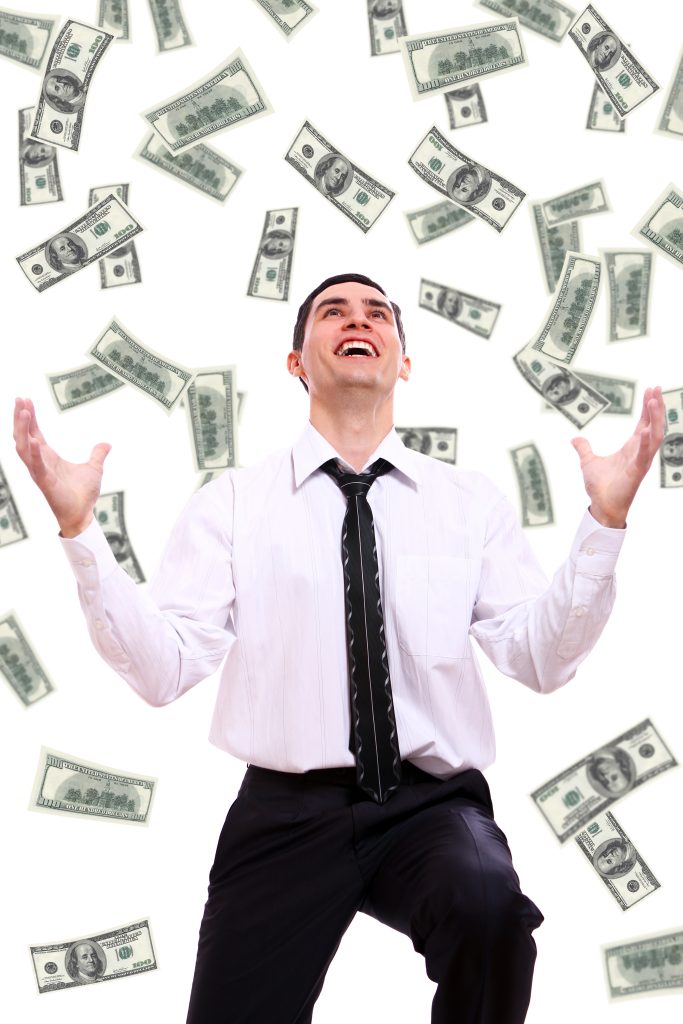
8. Solana congestion
It lasted 3 days with all green stats for us. We were so hyped when all of the sudden our transactions started to fail. What was happening? Solana congestion hit, causing around 70% of all transactions to fail. Needless to say, this affected us massively, just when we were starting to make money.
So, what did we do? We spent a week on transaction optimizations. We calculated computing budgets, set up fees, and searched for ways to improve our transactions. Honestly, it didn’t help much, so we had to wait for the congestion to clear up.
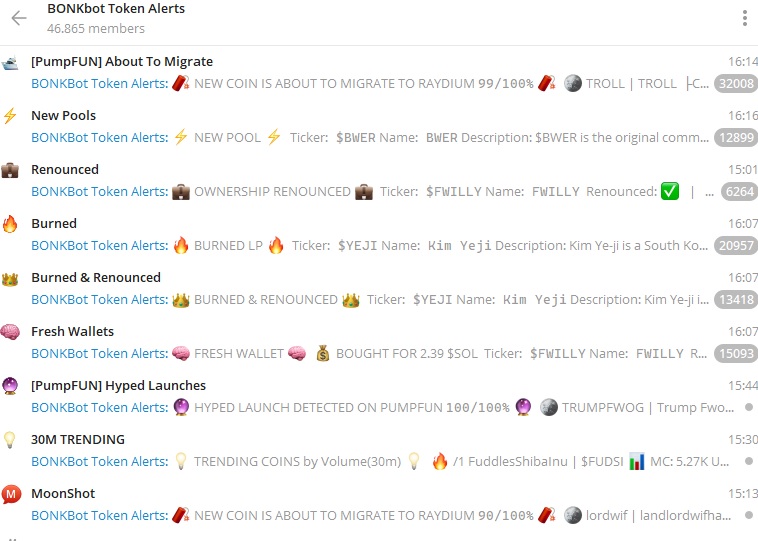
9. Telegram bot
We decided to introduce Telegram integration into our bot. By subscribing to Bonk Bot’s pool discovery, we could receive additional pool data such as whether the tokens are burned, if the pool liquidity is burned, the distribution of the tokens between holders, and other valuable insights.
Here’s what we did. We set up a bot on Telegram to subscribe to Bonk Bot’s pool discovery notifications. Through these notifications, we gathered extra details about the pools, including token burn status, liquidity burn status, token distribution, market cap etc. This information helped us refine our trading strategies and make more informed decisions.
By incorporating Telegram into our workflow, we added another layer of data to our bot’s evaluation process, hoping to improve our overall success rate.
10. Making money
The Solana congestion was finally over, and our bot was ready to shine. We had our blockchain discovery for new pools through Bonk Bot pool discovery in place, optimized blockchain transactions, and most importantly, a winning pattern. We were making money!
Our first successful bot, named Ziggy (thanks to its IPFS filter), was a huge success. It ran 24/7 on multiple instances across different locations worldwide, and it was profitable. We increased our stakes every 10 trades. Statistically, 8 out of 10 trades were profitable, so even with 2 failed trades, we were still in the green.
At the same time, we were testing other trading strategies, mostly focusing on pool evaluation with the new data from Bonk Bot pool discovery. Not wanting to keep all our funds in the same wallet, we added a new function to our codebase: once a certain amount was reached, the bot would transfer the funds to our Trezor wallet.
Everything was running smoothly, and Ziggy was our star player in the game of crypto trading. We also had a time to pimp our code a little bit and make it nicer, we even added some nicer terminal logs with a pino logger, just in case if something unexpected failed that we had logs for it.
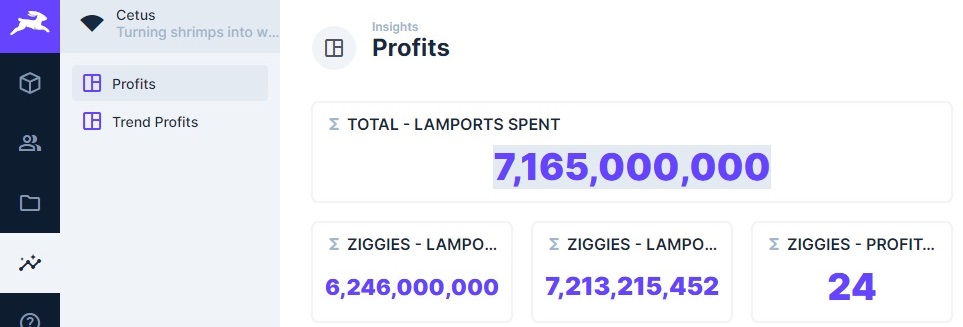
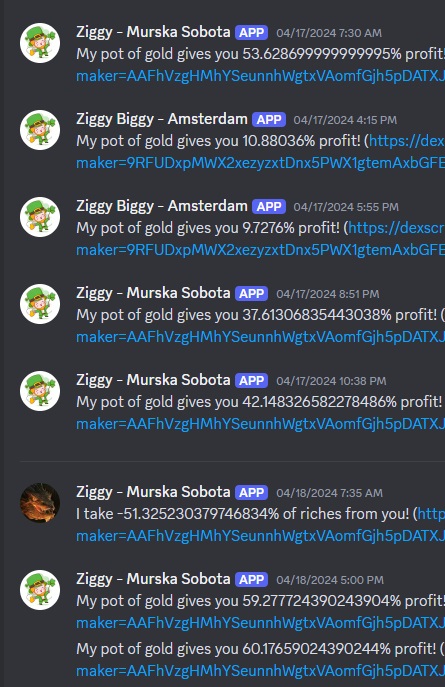
11. Discord bot
We didn’t rest on our success. Instead, we kept brainstorming ways to enhance our trading bot. One key improvement we implemented was a notification system to keep us informed about our earnings and losses. As big Discord users, we integrated a Discord bot to alert us whenever a trade was completed.
“Ding Ding! You have a new notification from Ziggy: a trade has been finished with a profit!”
This notification was music to our ears. There’s nothing quite like the thrill of your bot making money while you’re busy with something else. It doesn’t get better than this! 😀 Retirement here we come, but then …
12. Ending
For a few weeks, we were reaping the rewards with Ziggy, while also testing other bots. Though some showed promise, none matched Ziggy’s consistent profitability. Then, things took a turn for the worse. Scammers changed their publishing tokens, rendering our IPFS filter ineffective. Ziggy started incurring losses. We had to shut down Ziggy and all other bots, as they were all in the red.
Exhausted and out of funds, our excitement faded. We decided to stop developing our current project further in this direction that we choose. Instead we decided to risk some of our time a little bit more for 2 different approaches.
In the following week or two, we split our tasks in two groups. Filip started to work on Directus to try a long-term trading bot, where the strategy was to collect tokens that had been trading for a few days and, if they were still active on Raydium after a week or more, we would trade on them.
Meanwhile I spent my time on several scripts to validate token creators. I checked if they were unique and if they received initial funds from reputable crypto markets like Binance, Coinbase, and Kraken, rather than from fake wallet addresses. I also created scripts to verify if the pool liquidity was truly burned, as Bonk bot’s pool discovery was often inaccurate due to scammers’ sophisticated methods. Sadly all of the new evaluations didn’t make any changes.
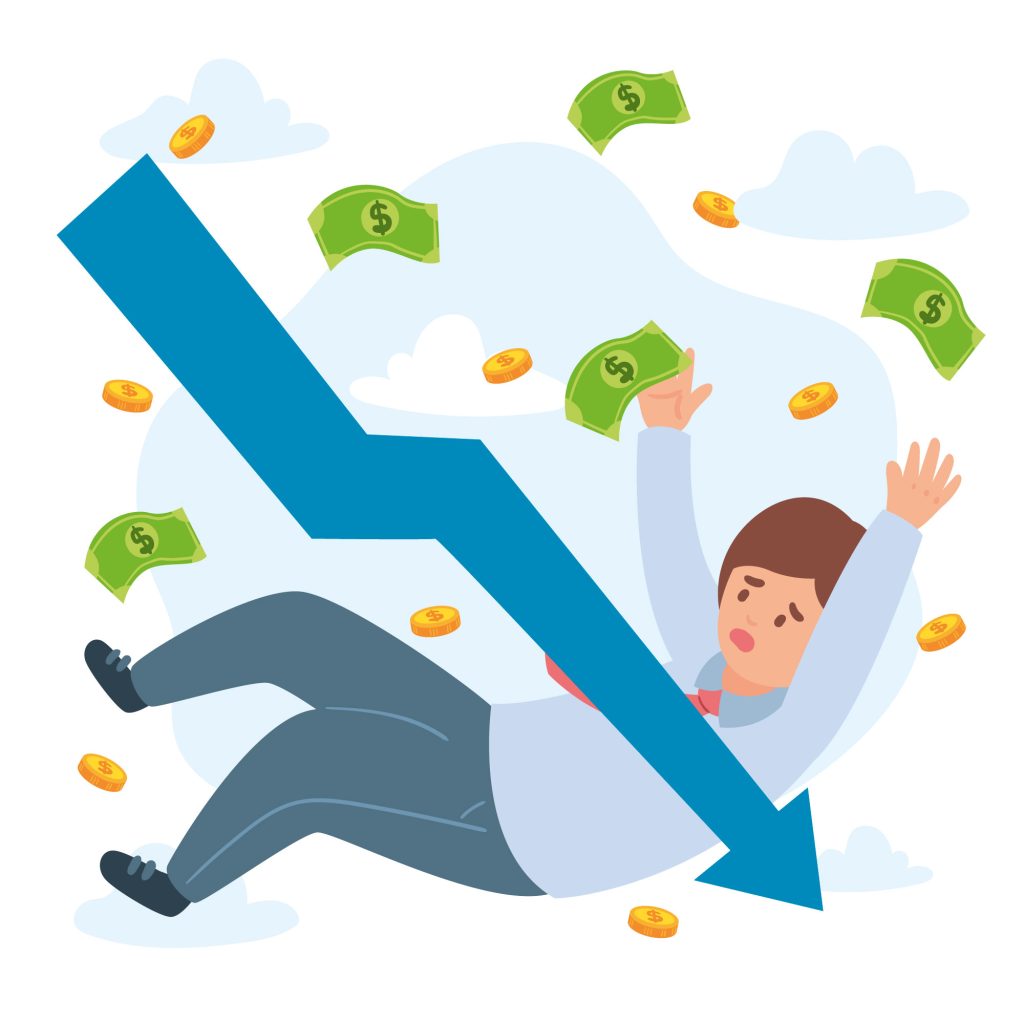
13. Conclusion
We invested a significant amount of time working on it full time for the past 6 months also over the weekends, having day meetings, todos board, the whole project management. We put so much effort into developing one of the most intriguing and challenging projects we’ve ever undertaken. The journey was filled with excitement as we delved into various technologies, from trading algorithms to sophisticated validation scripts and blockchain analytics.
For a while, we enjoyed the thrill of being in profit with Ziggy. It felt like a major victory. However, the scammers outsmarted us, leading to substantial losses. Despite the financial setback, the project was an invaluable learning experience. We gained deep insights into cryptocurrency trading, security measures, and the ever-evolving tactics of scammers.
Our frustration with scammers grew as their methods continually disrupted our progress. Despite this, the knowledge and skills we acquired throughout this venture were priceless. While the project ultimately ended in a loss, we haven’t completely ruled out the possibility of revisiting it in the future. With more experience and perhaps new strategies, we might find a way to overcome these challenges and succeed where we previously struggled.
Do we regret it? Certainly not, except for one thing that still sits in our minds. We should have been greedier when Ziggy was working, instead of buying tokens in amounts under 0.3 WSOL. We should have increased our buy amount to at least 1 WSOL or more. However, we were a bit afraid that this would disrupt the market since, in the beginning, the market cap and liquidity were really small, and we were competing with other bots that could sell the tokens before us.
That being said, we hope our experiences can provide valuable insights and help you navigate the complex world of cryptocurrency trading. Feel free to drop us a message for more information or if you would like to have access to the whole script.